Recommendation Script Functions
You can use the Dynamic Yield recommendation engine to return the results of a recommendation without Dynamic Yield rendering it, using a client-side API. This enables you to control how to display the recommendation instead of serving the recommendation in the HTML through a recommendation campaign. It also enables you to add real-time filters to get real-time results based on data obtained within the session (for example, show products priced higher than the currently viewed product or present products based on the visitor's explicit selection).
The results are returned as a set of products in JSON format.
Before serving recommendations via client-side API...
While the client-side API enables you to implement recommendations without a campaign, we still recommend that you implement it within a variation. This way, you can later run tests, use a control group to measure impact, and target different algorithms for different audiences without writing code on your site.
Serving recommendations via script functions
Create a strategy to call via API
- Go to Assets › Strategies.
- Create a recommendation strategy, and turn on the Use via an API call (advanced) toggle in the Strategy settings.
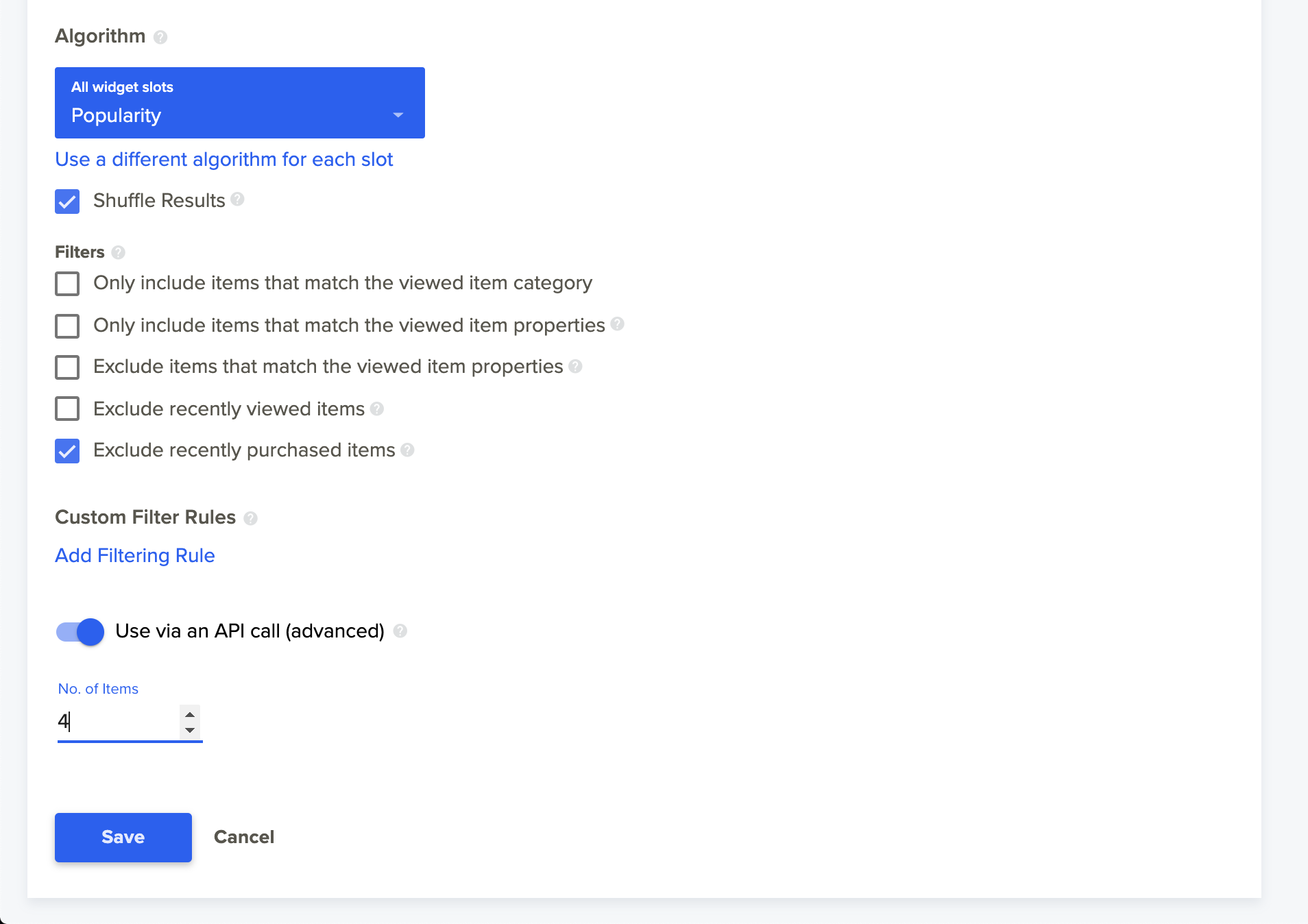
- Enter the number of items you want the API to return (usually, the number of items you plan to render).
- Save the strategy.
- In the Recommendation Strategies list, from the More Options menu, select Embed Code and then copy the API snippet.
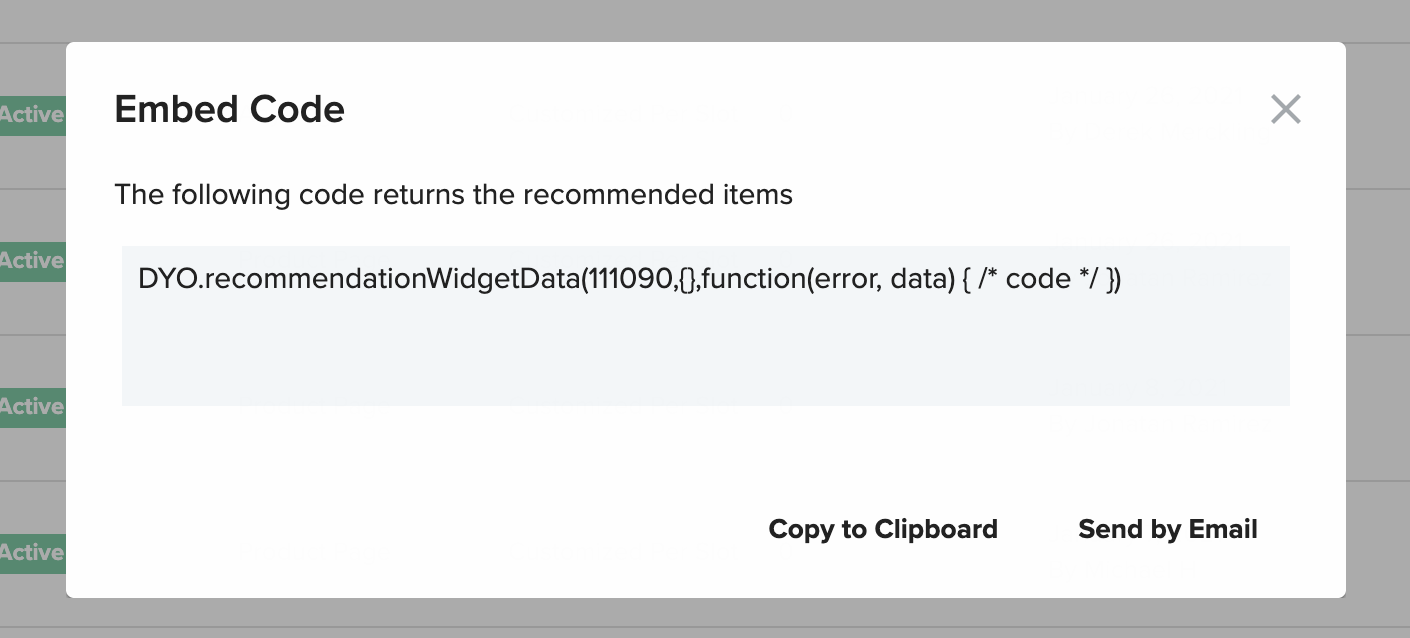
- Edit the recommendationWidgetData API as required. For details, see the following sections. You can also use this API in addition to the Return Recommendation real-time Filter Data API to add real-time filter rules to filter the results based on data obtained within the session.
- Paste the code into a Custom Code campaign to execute it for the relevant users.
- Implement reporting clicks to see recommendation performance using the recommendations.registerElements API. See API details in the following sections.
Render recommendations or dynamic content
Render the recommendation defined by a name or ID. This is only applicable for campaigns with Location set to Embed with code:
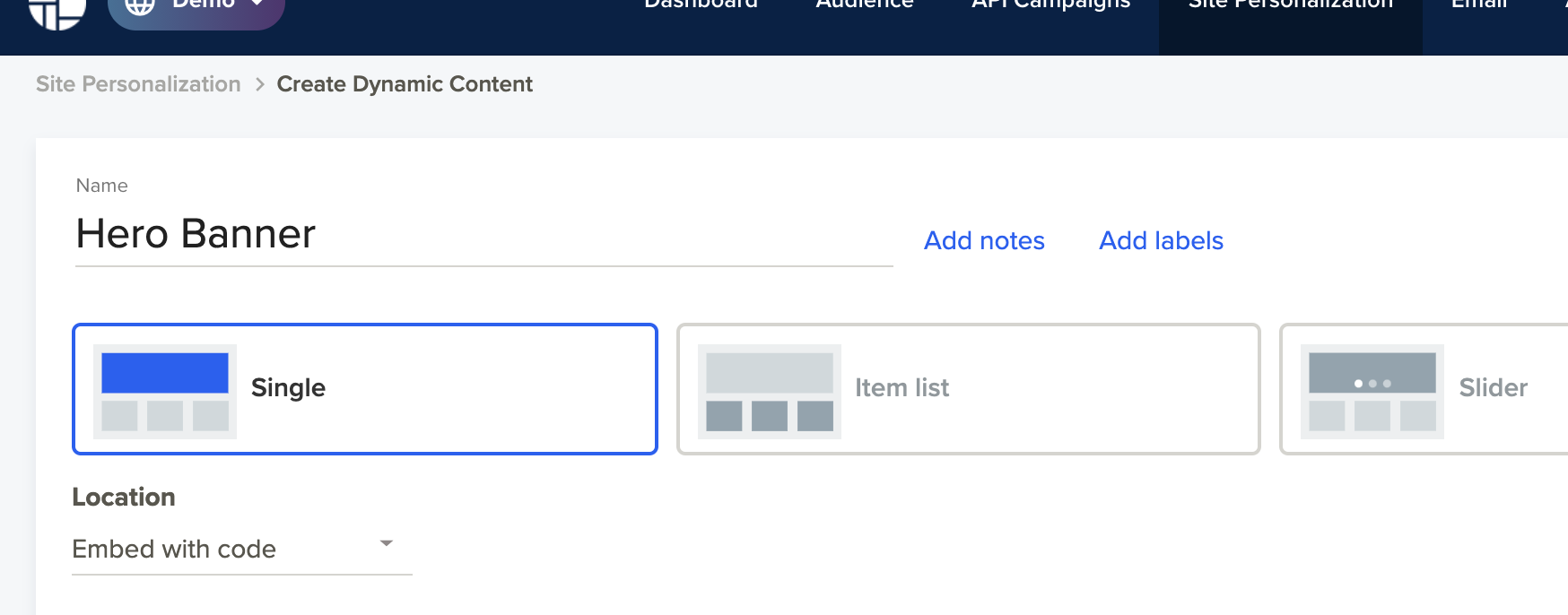
Return recommendation widget data
The results are returned as a set of products in JSON format. This API also requires you to track the recommendation widget as detailed in the following section.
Syntax:
DYO.recommendationWidgetData(id, opts, callback)
Parameters:
- id: The ID of the strategy to use when rendering the recommendation.
opts: Optional parameters- context: The page context is taken from the current page unless you specify a different context here.
- maxProducts: Overrides the “number of items” value from the Strategy configuration screen.
- exclude: An array of SKUs or Group_IDs to exclude from the recommendation results. This is often used to prevent displaying the same item more than once on a page that has multiple recommendation widgets. (This option is not available for the recently viewed strategy which doesn't use this item removal function)
- realtimeRules: Add real-time filter rules in order to enable real-time results based on data obtained within the session. Use-case and real-time filter data information can be found in Return Recommendation Realtime Filter Data.
- distinct: When sending 'true', you will not receive SKUs that are part of other recommendation widgets on this specific page.
- callback: Callback function to execute once recommendation data is returned.
Call example:
// pass product context manually to request
DYO.recommendationWidgetData(12345, {
exclude: {
'group_id': ['127829', '1217317', '1247581']
},
context: {
'lng': 'en_GB',
'type': 'PRODUCT',
'data': ["1253851-001"]
}},
function(err, data) {
if (err) {
//error handling
}
var rcomData = data;
});
Response example:
{
"wId": 3197,
"name": "TOP_N", // strategy name
"expData": {
"varId": null,
"expId": null
},
"fId": 543,
"fallback": true, //at least one of the items used a fallback
"slots": [
{
"item": {
"entity_id": "330367",
"color": "1349",
"product_url": "......html",
"color_prod_img": "https:.....3.jpg",
"publish_time": "2019-07-05T03:22:08",
"flag_img_url": "NONE",
"size_label": "38",
"color_image": "https://tropicalflowerdress.......jpg",
"size": "1899",
"category": "dresses",
"related_colors_label": "string",
"title": "great flower dress",
"related_colors": "619,628,3064,3070,3133",
"color_label": "flower",
"item_group_id": "620",
"category1": "women",
"sku": "6510120",
"description": "the best tropical flower dress in the universe",
"price": 199,
"in_stock": true,
"categories": [
"women",
"dresses"
],
"dy_display_price": "199",
"name": "tropical flower dress",
"url": "https://www.tropicdresses........html",
"category4": "",
"flag": "",
"tier_prices": "NONE",
"thumbnail_url": "https://tropicdresses......jpg",
"image_url": "https://tropicdresses......jpg",
"keywords": [
"women",
"dresses",
],
"group_id": "33112327",
"quantity": "2"
},
"fallback": true, //this item used a fallback strategy
"strId": 1,
"md": {}
},
{
"item": {
"entity_id": "330368",
"color": "1349",
"product_url": "......html",
"color_prod_img": "https:.....4.jpg",
"publish_time": "2019-07-06T03:21:00",
"flag_img_url": "NONE",
"size_label": "38",
"color_image": "https://tropicalflowerpants.......jpg",
"size": "1899",
"category": "pants",
"related_colors_label": "string",
"title": "great flower pants",
"related_colors": "619,628,3064,3070,3133",
"color_label": "flower",
"item_group_id": "534",
"category1": "women",
"sku": "6510145",
"description": "the best tropical flower pants in the universe",
"price": 89,
"in_stock": true,
"categories": [
"women",
"pants"
],
"dy_display_price": "89",
"name": "tropical flower pants",
"url": "https://www.tropicpants........html",
"category5": "",
"flag": "",
"tier_prices": "NONE",
"thumbnail_url": "https://tropicpants......jpg",
"image_url": "https://tropicpants......jpg",
"keywords": [
"women",
"pants",
],
"group_id": "33112347",
"quantity": "1"
},
"fallback": false, //this item used the chosen strategy
"strId": 1,
"md": {}
},
]
}
Track the recommendation widget
To track a recommendation widget that you manually added to your site using the recommendationWidgetData API, you must make sure Dynamic Yield can track the widget as well. The JSON results of recommendationWidgetData are used to configure this tracking.
Syntax:
DYO.recommendations.registerElements(el);
Usage:
- Add the following attributes to the DOM element that contains the recommendation widget:
<div data-dy-widget-id="WIDGET_ID_HERE" data-dy-feed-id="FEED_ID_HERE">
<div data-dy-product-id="PRODUCT_SKU_HERE" data-dy-strategy-id="STRATEGY_ID_HERE">...</div>
<div data-dy-product-id="PRODUCT_SKU_HERE" data-dy-strategy-id="STRATEGY_ID_HERE">... </div>
. .
</div>
- widget id: the wId from the JSON response. This is the ID of the strategy.
- feed id: the fId from the JSON response.
- strategy id: the strId from the JSON response (can be found under each "slot"). This represents the strategy type of each slot.
- product id: the sku of each product from the JSON response.
- Run the following API call:
DYO.recommendations.registerElements(el);
Where el is the DOM element with the widget ID attribute (data-dy-widget-id).
Show all recommendation widget responses
Presents all products returned by all recommendation widgets on the page.
Syntax:
DYO.recommendations.getLoadedWidgets()
The response is an array of Return Recommendation Widget Data results for all recommendation widgets returned on the page.
Updated 7 months ago